Roshan Nayak
Software Engineer
© 2021 All rights reserved.
Top 100 Automation Testing Interview Questions
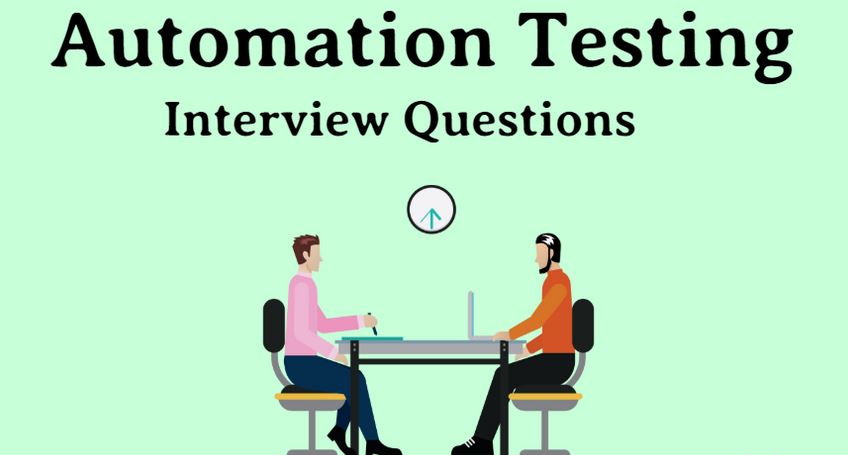
Java-Basic
- Types of polymorphism
- Difference between override and overload
- Methods that cannot be overloaded
- Static block and instance block
- Difference in Static and non static
- Encapsulation and common use cases
- Abstract vs interfaces
- Inheritance in java
- Methods that cannot be overloaded
- Type casting in java
- String buffer and string builder
- Why string is immutable in java
- How to handle exceptions
- Can I write try catch without the catch block
- Difference between throws and throw
- Use of iterator in java
- Difference in Final, finally and finalize
- Boxing and unboxing in java
- Increment and decrement operation
- Variable Args
- This and super keyword in java
- Issues during Switich case without break
- Upcasting and downcasting
- Baseclass of all class in java
- Baseclass of error and exceptions
- Access specifiers
- Continue and break statement
- Can main method return any value?
- Can we overload main method. What happens when overloaded
- How to execute and statement before main method
- Difference between == and equals()
- Can user declare constructor as final
- Can we cast any other type to Boolean data with type casting.
- Does java compile if user use ‘static public void’ instead of ‘public static void’?
- Can we use this() and super() in a constructor?
- Can we create object of abstract class?
- Can we create reference for an abstract class?
- Can we declare a class as static?
- What is instance Of keyword
- What’s the load factor of HashMap?
- How to prevent a class from being sub classes?
- Final variable, final method and final class
- Ways to create a string variable.
- What is gc() – garbage collector
- Subclass and innerclass
- Infinite loop in java
- How to make copy of an element
- Checked and unchecked exceptions
Java Collection
- Classes inside List interface, Set interface, Map Interface
- Arraylist vs Linkedlist
- Arraylist vs array
- Arraylist vs vector or stack
- Which class of List Interface to be used if user have more insertions and deletions
- Which class of List Interface to be used if user have more retrieval
- Set Interface: HashSet, TreeSet, SortedSet
- Map – HashMap, HashTable, TreeMap, LinkedHashMap.
- Stack and Queue
- How to maintain insertion order in Set, List and Map
- How to sort elements in ascending order in Set and Map
Java Programs
- String reverse
- String Palindrome
- String Anagram
- Find occurrences of characters in a string
- Find the count of Capital and Small letters in a string
- Remove duplicate characters from string
- Swap to numbers without temporary variable
- Reverse number
- factorial
- Fibonacci
- Count number, alphabet and special characters
Selenium
- Difference in driver.get() and driver.navigate().to()
- Navigation methods in selenium
- What is POM
- Implicit wait
- Explicit wait- use of explicit wait, how to ignore exceptions using explicit wait
- Fluent wait
- Examples for expected conditions in explicit wait
- Default timeout of pageLoadTimeout.
- Use of desired capabilities
- Use of chromeOptions, firefoxOptions ,ieOptions, etc.
- How to disable notification in selenium.
- Difference between getText() and getAttribute()
- How to extract css property value
- Difference between driver.close() and driver.quit()
- How to handle dropdown elements with select tag and without select tag.